After talking about inspector attributes in part 2, it is time to switch our focus to the scene view. Most of editor scripting in Unity is changing and dealing with the editor by adding buttons, making UI changes or creating new windows, however, there is a small part of the editor API that allows you to work with the scene view, and those are Gizmos.
Gizmos are all these little UI elements and graphics present all over the scene view, all the different icons on objects, like the sun and camera icons, the outline around selected objects and the collider outline are all in reality gizmos.
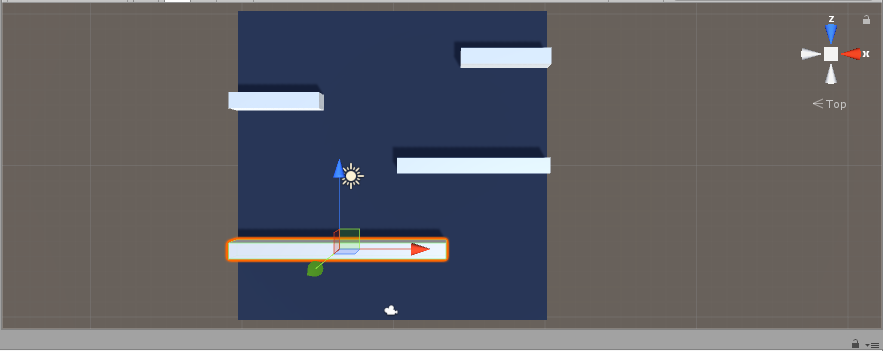
Gizmos are all around us
Gizmos are superb visualization tools that, used correctly, can can provide a lot of information about something in the game in an instant. Combined with Handles (see Part 4!) they can be made interactable, which means we can create selection and control gizmos for say parts of your complex objects containing many children.
For today’s example, we will be using gizmos to help us visualize a very simple path tool.
We want to create a tool that allows us to create a path using waypoints that can be moved in the scene view while also getting a visualization of the path between said waypoints.
Another thing is that we don’t want the waypoints to be actual game objects, since those carry unnecessary overhead. Our waypoints will simply be an array of Vector3
representing the world positions of each waypoint.
1using UnityEngine;
2
3public class Path : MonoBehaviour
4{
5 public Vector3[] waypoints;
6}
This will give us a list of position variables to work with, but the problem is that we can’t see the waypoints in the scene nor can they be moved easily. Today we will work on visualizing the waypoints and path using Gizmos.
All you need to use gizmos is the UnityEngine
namespace and one of these methods: OnDrawGizmos
or OnDrawGizmosSelected
.
The first method will show your gizmos as long as the object is active and the component is expanded, while the second method requires the
object to be active and selected in the hierarchy. For now we will just use the OnDrawGizmos method, which note is called every update.
First lets visualize where the waypoints are by drawing spheres on their positions:
1void OnDrawGizmos()
2{
3 Gizmos.color = Color.green;
4 for (int i = 0; i < waypoints.Length; i++)
5 {
6 Gizmos.DrawSphere(waypoints[i], 0.5f);
7 }
8 Gizmos.color = Color.white; //Reset so other Gizmos aren't affected
9}
All gizmo related stuff are done via the Gizmos class. On line 3 the color is set to green, this makes all gizmos created after it have the new color.
Then we call the DrawSphere
method which takes a position and a radius for the sphere, and finally we reset the color.
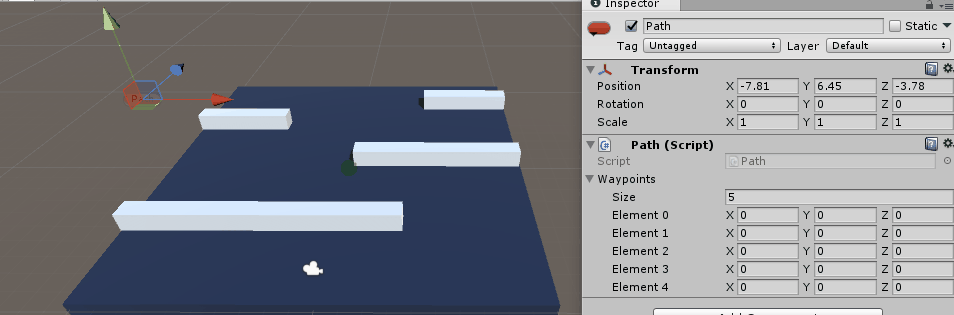
Sphere Gizmos in the scene
With very little code we are already starting to see nice results, but there are still a few things we can do to improve this. One obvious thing is showing the actual path, after all this is a path system, so what we will do now is to draw a line between each waypoint and the point after it.
1void OnDrawGizmos()
2{
3 Gizmos.color = Color.green;
4 for (int i = 0; i < waypoints.Length; i++)
5 {
6 Gizmos.DrawSphere(waypoints[i], 0.5f);
7
8 //If it is the last point don't try to draw a line
9 if (i != waypoints.Length - 1)
10 Gizmos.DrawLine(waypoints[i], waypoints[i + 1]);
11 }
12 Gizmos.color = Color.white;
13}
The DrawLine
function is very simple to use, pass it start and end points and you are done!
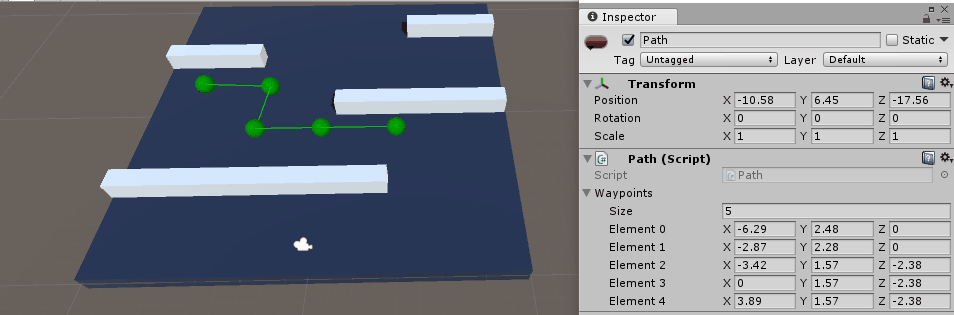
Lines between spheres (Play with Gizmos.Color to give lines a different shade)
We have only seen two draw functions so far, but there are a few more very similar functions that allow the drawing of things like cubes, wireframes, and meshes which you can see here.
We are almost done, however this path tool is not very usable due to the fact that we can’t move waypoints easily using something like the translation tool. It would also be nice if we can number the waypoints in the scene.
Those things can’t be done with gizmos, but Handles allow us to have interactivity, but I’ll leave that for Part 4.